Learning Blockchain Programming: Choosing a Platform & Languages
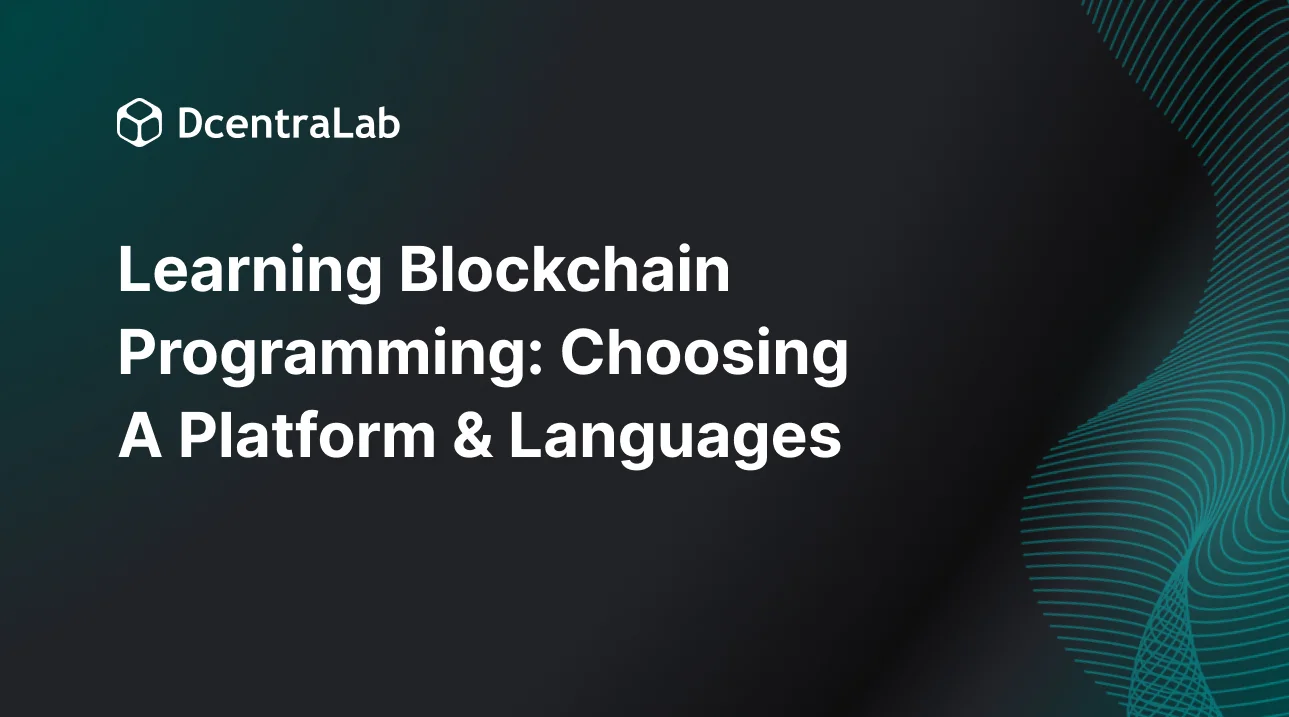
tl;dr
- Blockchain is a decentralized, secure digital ledger storing transactions in linked blocks.
- Key blockchain languages: Solidity (EVM-compatible smart contracts), Rust (Solana), Haskell (Cardano).
- Development tools: Remix, Hardhat, Truffle (IDEs); Mocha, Chai, Jest (testing); Git/GitHub (version control).
- Beginners can learn Solidity through platforms like CryptoZombies and practice with tools like Token Factory.
Understanding Blockchain Basics
A blockchain is a decentralized digital ledger that records transactions across a network of computers in a secure and immutable way. Each transaction is stored in a block, which links to the previous one, forming a chain.
Blockchains work by using cryptographic algorithms to validate and secure transactions. Once verified, blocks are added to the chain, and the data is distributed across all network participants, ensuring transparency and resistance to tampering.
A blockchain can be public, private, or consortium-managed. Public blockchains are open to everyone, private blockchains are restricted to specific users, and consortium blockchains are managed by an organization group.
Choosing the Right Blockchain Platform
Different blockchains cater to different audiences and can influence the success of your web3 developer career. As such, it’s always best to research what chain suits you and your project best. Here are a few of the more popular choices.
Bitcoin and the Lightning Network
Programming on Bitcoin focuses on leveraging its secure, decentralized blockchain for apps, while the Lightning Network enhances its capability by enabling fast, low-cost micropayments. Bitcoin's design limits the creation of complex dApps, unlike Ethereum, but innovations like Ordinals (NFTs on Bitcoin) have emerged.
Lightning Network Apps (LApps) address Bitcoin's scalability and dApp limitations, offering solutions for instant transactions. Developers build LApps by setting up Bitcoin and Lightning nodes, integrating tools like BTCPay Server, and simulating environments with tools like Polar. Although more constrained than Ethereum's ecosystem, LApps enable efficient micropayment solutions for Bitcoin-based applications.
Ethereum
Programming on Ethereum involves developing smart contracts, primarily using Solidity, a language tailored for Ethereum and other EVM-compatible blockchains.
Solidity enables developers to create DApps by leveraging features like inheritance, encapsulation, and polymorphism. Key functionalities include public and private data members, contract-based structures akin to classes, and predefined variables like msg, tx, and block.
Ethereum’s expansive community supports robust development, and its EVM compatibility allows smart contracts to extend to Layer 2 solutions like Base and other Layer 1 chains. This ecosystem fosters innovation across DeFi, NFTs, and beyond.
Solana
Programming on Solana leverages its high performance and low fees, making it a rapidly growing blockchain. Development primarily uses Rust, a language known for its memory safety and efficient concurrency.
To create Solana dApps, developers write and compile Rust-based code, generating a .so file and a keypair file for deployment. Key tools include the Solana CLI, Rust, and IDEs like Visual Studio Code. While Rust is dominant, Solana also supports C, C++, and Solidity (via Neon EVM). For client-side interactions, SDKs like @solana/web3.js in TypeScript or libraries in Python, Java, and other languages enable dApp creation.
Polkadot
Programming on Polkadot involves using Rust to develop custom blockchains and decentralized applications within its interoperable network. Developers typically create parachains or substrates, leveraging Rust’s performance and safety features to build scalable, secure solutions.
Despite Polkadot's advanced architecture and focus on interoperability, its community and ecosystem are smaller than Solana’s. As a result, Rust developers may prioritize Solana for its broader opportunities and support network.
Cardano
Programming on Cardano combines the benefits of its unique UTXO architecture with the flexibility of a smart chain, allowing developers to build secure, scalable dApps. Cardano’s ecosystem supports multiple programming languages, with Haskell and Plutus as the core.
Haskell underpins Cardano’s architecture, emphasizing formal verification and high-assurance code for critical systems. Plutus, a Haskell-based language, is optimized for writing on-chain code, compiling to Untyped Plutus Core (UPLC) for execution. This approach ensures robust security and precision.
With a large, loyal community, Cardano provides a strong foundation for developers seeking a balance between Bitcoin’s and Ethereum’s strengths.
Essential Programming Languages for Blockchain Development
As we’ve seen, different blockchains support different programming languages. Here’s a slightly deeper dive into what you need to know.
Solidity
Solidity is a high-level, contract-oriented programming language tailored for developing smart contracts on Ethereum and other EVM-compatible blockchains. It is statically typed and supports advanced features like inheritance, libraries, and custom data types, with syntax resembling JavaScript and C, making it accessible to many developers.
Smart contracts in Solidity consist of functions and state data that reside at specific blockchain addresses, enabling interaction between contracts and the execution of complex tasks. Solidity supports diverse data types, including integers, arrays, mappings, structs, and custom enumerations, alongside functions with visibility modifiers (public, private, internal, external) to manage access control.
Development tools such as Remix IDE, Truffle Suite, and Hardhat simplify the coding and deployment process, with the Solidity compiler (solc) translating code into bytecode for execution on Ethereum. Security is paramount due to the irreversible nature of deployed smart contracts, which often manage valuable assets. Developers must also optimize their code for gas efficiency, reducing transaction costs.
Rust
Rust is a modern systems programming language focused on safety, performance, and concurrency. It ensures memory safety without garbage collection, addressing issues like null references and dangling pointers through its unique ownership system. This system assigns each value a single owner and a specific scope, simplifying memory management.
With syntax similar to C++, Rust is approachable for many developers, and its strict compiler provides detailed error messages to encourage safe coding practices. Cargo, Rust’s package manager, streamlines dependency management and project builds, while its robust standard library offers APIs for common programming tasks. Rust’s design supports safe concurrency, preventing data races, and uses the Option type to handle absent values, eliminating null or undefined states. The language also includes powerful pattern matching for effective data handling.
Supported by a growing community, extensive documentation, and resources like The Rust Programming Language book, Rust provides a solid foundation for new learners.
Haskell
Haskell is a purely functional programming language that emphasizes immutability and treating computation as mathematical functions. It features static typing, where the compiler checks types at compile-time, reducing runtime errors. Haskell uses lazy evaluation, meaning expressions are evaluated only when their results are needed, enabling efficient processing of infinite data structures. Its syntax supports pattern matching for defining functions and handling cases, as well as guards for conditional execution. Arithmetic operations use standard symbols, while string concatenation uses the ++ operator.
Haskell supports common data types like Int, Float, Bool, and lists, alongside higher-order functions and type inference, enhancing flexibility and conciseness. The Glasgow Haskell Compiler (GHC) is widely used, complemented by GHCi, an interactive environment for testing and debugging code. Dependency management and project building are handled by Cabal or Stack.
Haskell is well-suited for applications ranging from numerical computation to symbolic processing. Beginners often start with resources like Learn You a Haskell for Great Good! to explore the language’s concepts.
Key Development Tools and Frameworks
Blockchain development relies on various tools and frameworks to streamline the process and ensure robust applications. Integrated Development Environments (IDEs) like Remix, Hardhat, and Truffle provide essential features for smart contract development. Remix is browser-based and ideal for beginners, offering a simple interface for writing, deploying, and testing contracts. Hardhat and Truffle are more advanced, supporting extensive project management and integration with local blockchain environments.
Testing frameworks are crucial for ensuring the reliability of blockchain applications. Tools like Mocha, Chai, and Jest help developers write unit and integration tests for smart contracts. Truffle also includes built-in testing capabilities, while Hardhat allows automated test execution using JavaScript and TypeScript.
Debugging tools simplify identifying and resolving errors. The Remix IDE includes an in-depth debugger for analyzing transaction behavior, while Hardhat offers stack traces and error messages tailored for blockchain projects. Tools like Ganache create a local Ethereum blockchain to simulate and debug complex interactions without incurring network costs.
Version control systems, such as Git, are indispensable for managing code changes in collaborative environments. Platforms like GitHub or GitLab enable teams to track development progress, handle contributions, and maintain project history, ensuring streamlined collaboration in blockchain development.
Building Your First Blockchain Application
Building your first blockchain application starts with learning Solidity, the programming language for Ethereum smart contracts. Beginners can explore platforms like CryptoZombies, an interactive tutorial that teaches how to create simple dApps through gamified lessons.
Once comfortable, developers can experiment with tools like Token Factory to mint custom tokens, gaining practical experience with Ethereum’s ERC standards.
Additionally, exploring payable functions allows developers to handle transactions and integrate payments into their dApps.
The Future of Blockchain Development
The future of blockchain development is promising, with evolving trends in Web3, decentralized finance, and NFTs. New developers should gain practical experience by seeking Web3 jobs via platforms like LinkedIn or web3.career.
Staying updated on industry trends through Reddit, Twitter, or Farcaster is also essential for success.